
To save the record to the database, you can call the save method:

You can confirm that it isn't saved by running Those will be set when the record is saved to the database, which it currently isn't (if you typed exit right now, nothing will have been stored in the database). Notice that three of the six properties have been set, while the other three haven't. The second line is the value of our variable p: a new Person object. When we created the Person model, a file app/models/person.rb was created it looks like this: This is the piece that you'll actually interact with from your Rails controllers. Now that we've set up the database, we're ready to look at the other part of our model: the Active Record class. Last note on migrations: here's a list of the supported types that you can use in your migration classes: We can't go into them all in this tutorial, but if they look like something you'll need, check out the migration docs. Yes, I've forgotten this my fair share of times, and wondered what was going on, only to realize that the table I was trying to work with didn't even exist yet.īesides create_table and add_column, there are a bunch of other methods that you can use in your migration files. = DoStuff: reverted (0.0140s) =Īnd now, the migration we wrote from scratch has been undone.Īn important note here: if you're relatively new to Rails, you might forget that migrations aren't run automatically when you create a new model. Now, let's undo the last migration we ran. The first migration created the people table the second created the nothing table and added the column. You can see from the output exactly what was done. It will have the up/ down methods by default, but do ahead and replace that with a single change method. Let's check it out.Ĭlass CreatePeople _do_stuff.rb. You should be comfortable with the migration file syntax, because you'll often want to adjust the code. The first file is your migration file (of course, the timestamp will be different) the second is your Ruby class. The important bits are this:Ĭreate db/migrate/20130213204626_create_people.rb So, what's this actually do? You should see some output, explaining that. For age, we say that it should be an integer. For first_name and last_name, we don't specify a type, so it defaults to a string. We're saying we want this model to have three fields: first_name, last_name, and age. The third one gives Rails a little more information, so it can do a little more work for us. The first two lines here do the same thing rails g is just a shortcut for rails generate. Rails g model Person first_name last_name age:integer Actually, the rails generate command is pretty flexible: all the following commands work: From the command line, we'll create a model for, say, a Person. If you've got that installed, you can start by creating a new Rails app.Īs you might expect, since Active Record interacts with the database, it is the M in Rails' MVC: models. I'm using Rails 3.2.12, the latest version as I type this. While it is technically possible to use Active Record outside of a Rails app, nine times out of ten you'll be using it within a Rails app, so that's what we'll do here. So, that's what Active Record is let's dig deeper and see how it all works. Same code, different database, which is pretty cool, in my opinion. Now, when you deploy, Heroku will run those same migrations (using the PostgreSQL adapter). All you have to do is add this to your Gemfile: However, let's say you're deploying to Heroku, which uses PostreSQL. For example, Rails uses SQLite locally when you're developing. The cool part is that it doesn't matter what database you're using: Rails can handle pretty much all of 'em. These migrations make the actual changes to the database.
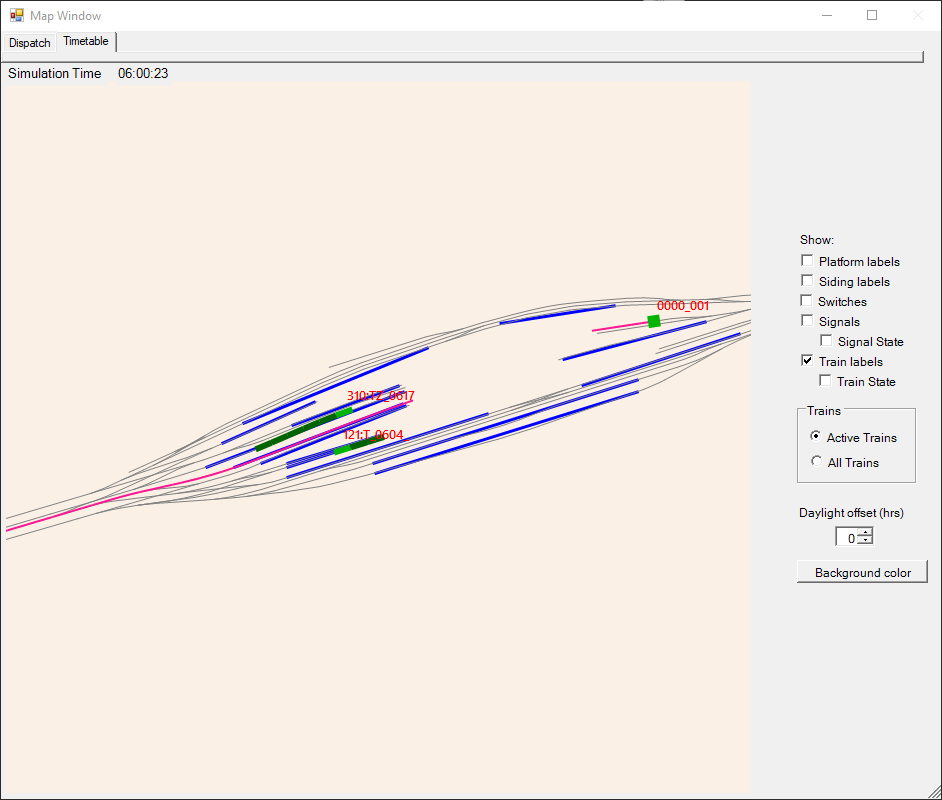
When you need to make changes to the database, you'll write Ruby code, and then run migrations, which we'll review soon.
#Rails command see all active tables code#
This means it's a layer of Ruby code that runs between your database and your logic code. In this tutorial, we'll dive into Active Record and see what it can do for us! Rails uses an ORM in the form of Active Record.
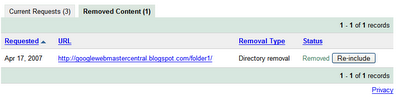
More recently, however, back-end frameworks are leaning toward using Object-Relational Mapping (ORM) this is a technique that lets you manage your database in the business logic language that you're most comfortable with. In the past, to build a web application, you required the skills to code in your business logic language and your database language.
